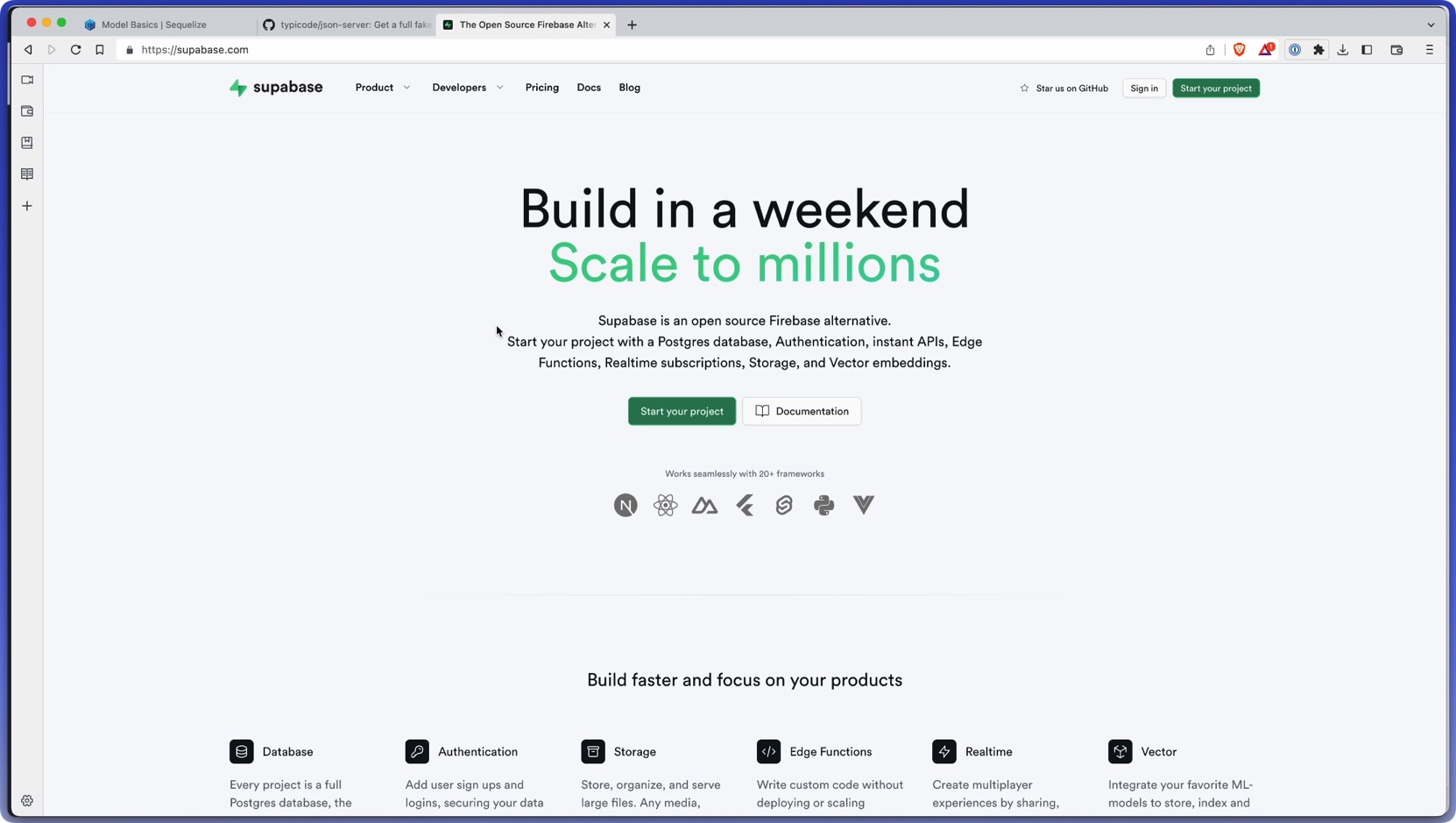
Authorization, Part 1
Buy or Subscribe
You can access this course in just a minute, and support my efforts to rid the world of crappy online courses!
We can't escape it: we need a database! We have lots of choices but I'm going to go with what I would really do, so here we go!
Considerations
How do we want to store our data and, more importantly, how should we get started?
- Keep ignoring it and use something like
json-server
- Use a hosted system that's easy, like Supabase or Firebase
- Roll up our sleeves and start building our models
I don't like the idea of writing code I know I'll throw away, so maybe we go with the 3rd option. Which leaves the question...
Why Not Firebase or Supabase?
Short answer: these are great choices which I'll get into later, but only if you understand:
- They replace your entire backend and API
- Security rules! So easy to mess up.
- They also replace your application state, in some ways (if you go full realtime)
My User Code
I have some code ready to go that I added to the project and we'll walk through
Sequelize Snippets
I use a few snippets for VS Code and here they are:
"Sequelize TEXT": {
"prefix": "mft",
"body" : [
"$0: DataTypes.TEXT,",
]
},
"Sequelize field": {
"prefix": "mf",
"body" : [
"$0: {",
" type: DataTypes.TEXT,",
" allowNull: false,",
"},",
]
},
"Sequelize money": {
"prefix": "mfm",
"body" : [
"$0: {",
" type: \"numeric(10,2)\",",
" allowNull: false,",
" defaultValue: 0,",
"},",
]
},
"Sequelize Model" : {
"prefix": "mod",
"body" : [
"const { Model, DataTypes } = require('sequelize');",
"class $1 extends Model{}",
"exports.init = function(sequelize){",
" $1.init({",
" $2: {",
" type: DataTypes.TEXT,",
" allowNull: false,",
" unique: true",
" },",
" }, {",
" sequelize,",
" underscored: true,",
" timestamps: false,",
" hooks : {",
" //https://github.com/sequelize/sequelize/blob/v6/src/hooks.js#L7",
" }",
" });",
" return { $1 };",
"}",
]
}